Using MagicRail API In Your Application
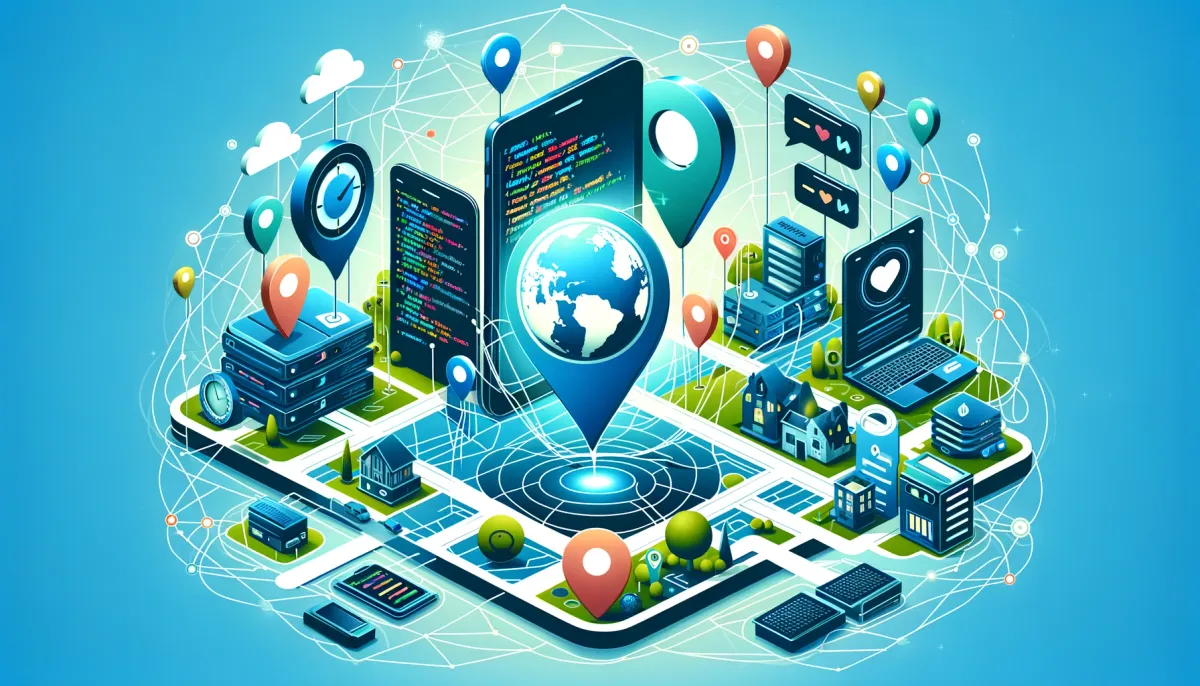
In the fast-moving world of app development, efficiency and functionality are key. If you're looking to boost your applications with advanced trip management and location-based services, the MagicRail API is your go-to solution. This tutorial will show you how to seamlessly integrate the MagicRail API into your Node.js projects, guiding you through authentication, creating trips, adding location data, and retrieving trip information. By harnessing these capabilities, you can significantly slash development time and costs, enabling you to deliver powerful features to your users more swiftly.
Prerequisites
Before diving into the tutorial, ensure you have Node.js installed on your system. You will also need axios
for making HTTP requests. If it's not already part of your project, you can install it using npm with the following command:
npm install axios
Step 1: Authenticating with MagicRail API
The first step involves authenticating with the MagicRail API to obtain an access token. Replace 'your_email@example.com'
and 'your_password'
with your own MagicRail credentials. Here’s how you can authenticate and obtain the token:
const axios = require('axios');
const username = 'your_email@example.com';
const password = 'your_password';
let token;
axios.post('https://api.magicrail.io/users/login', {
email: username,
password: password
}).then(response => {
token = response.data.token.accessToken;
console.log('Authentication successful. Token:', token);
}).catch(error => {
console.error('Authentication failed:', error);
});
Step 2: Creating a New Trip
Once authenticated, you can use the access token to create a new trip. Below is a function to generate a random string for use as an external ID (exId
) and then create the trip:
function generateRandomString() {
return Math.random().toString(36).substring(7);
}
const createTrip = async () => {
const exId = generateRandomString();
try {
const response = await axios.post('https://api.magicrail.io/trips/create', {
exId: exId
}, {
headers: { Authorization: `Bearer ${token}` }
});
console.log('Trip created. ID:', response.data.trip.tripId);
return response.data.trip.tripId; // Save this ID for future operations
} catch (error) {
console.error('Failed to create trip:', error);
}
};
Step 3: Adding Location Data to the Trip
After creating a trip, you can begin adding location data to it. Replace tripId
with the ID you obtained from the trip creation step:
const addLocationData = async (tripId) => {
try {
const response = await axios.post('https://api.magicrail.io/trip-data/create', {
latitude: 40.7128,
longitude: -74.0060,
elevation: 5.0,
time: '2023-01-01T12:00:00Z',
tripId: tripId
}, {
headers: { Authorization: `Bearer ${token}` }
});
console.log('Location data added:', response.status);
} catch (error) {
console.error('Failed to add location data:', error);
}
};
Step 4: Retrieving Trip and Location Data
Finally, you can retrieve details about your trip and its associated location data using the trip ID:
const getTripDetails = async (tripId) => {
try {
const tripResponse = await axios.get(`https://api.magicrail.io/trips/${tripId}`, {
headers: { Authorization: `Bearer ${token}` }
});
console.log('Trip details:', tripResponse.data);
const locationDataResponse = await axios.get(`https://api.magicrail.io/trip-data/${tripId}`, {
headers: { Authorization: `Bearer ${token}` }
});
console.log('Location data:', locationDataResponse.data);
} catch (error) {
console.error('Failed to retrieve data:', error);
}
};
Wrapping Up
Through this tutorial, Node.js developers can see how integrating the MagicRail API adds valuable trip management and location-based functionalities to their apps. By following these steps, developers can streamline their development process, save on costs, and enhance their app's offerings, ultimately providing a better experience for users.
Are you ready to simplify your application development? Join the growing number of developers who have streamlined their location data management with MagicRail. Sign up for a free trial today and see how easy and effective managing location data can be. Visit us at MagicRail.io to get started.